CreateProduct
Product data structure
Before creating a product via API, it is helpful to understand the content data structure.
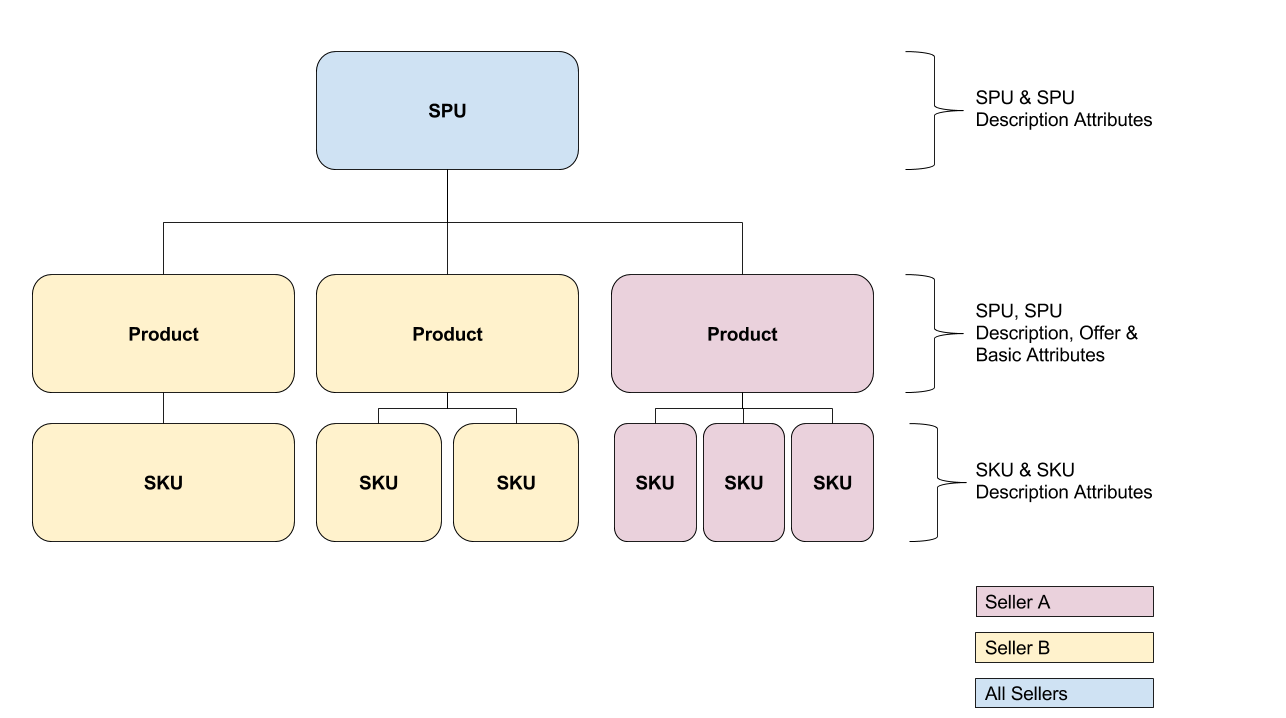
The highest level of an SKU is SPU. A product inherits attributes from the SPU, and the SKU inherits attributes from the PRODUCT.
The difference between an SKU and its product is the SKU ATTRIBUTES (which are mandatory) fields when you create your request.
Similarly, product attributes would be mandatory.
Definition
Use this call to create a new product. One item may contain at lest one SKU which has 8 images. This API does not support creating multiple products in one request.
Request URI: https://api.sellercenter.lazada.sg?Action=CreateProduct
Parameters
** Please note that SKU attributes are mandatory for all products created.
Field | Type | Description |
---|---|---|
Action | string | CreateProduct Name of the API that is to be called. Mandatory. |
Format | string | The response format, with XML as the default. Can be XML or JSON. Optional. |
Timestamp | datetime | The current time in ISO8601 format (e.g., Timestamp=2016-04-01T10:00:00+02:00 for Berlin). Mandatory. |
UserID | string | The ID of the user making the call. Mandatory. |
Version | string | The API version against which this call is to be executed. The current version is "1.0". Mandatory. |
Signature | string | The cryptographic signature, authenticating the request. You must create this value by computing the SHA256 hash of the request, using the API key of the user specified in the UserID parameter. Mandatory. |
Code sample
# CreateProduct cURL example. to run, update Timestamp and recompute Signature
#
url = "https://api.sg.ali-lazada.com/"
post
data-urlencode Action=CreateProduct
data-urlencode Timestamp=2016-07-18T11:11+0000
data-urlencode [email protected]
data-urlencode Version=1.0
data-urlencode Signature=9ade00fb4b9ab9ed1b8a4d189f1a13e1029edc25dd963235480ec69226fd9f39
Business parameters
The following tables list the parameters that are required for creating a product.
Name | Type | Description |
---|---|---|
Product | subsection | The product data node. Mandatory |
PrimaryCategory | integer | The ID of the primary category for the product. To get the ID for each of the system's categories, call GetCategoryTree. Mandatory. It's optional if 'AssociatedSku' is provided. |
SPUId | integer | The ID of the SPU. Optional |
AssociatedSku | string | The unique identifier of a product that is already in the system, with which this product should be associated. Optional |
Attributes | subsection | All common attributes of products. Mandatory. It's optional if 'AssociatedSku' is provided. |
Skus | subsection | An array contains at least one SKU. Mandatory |
You can use 'AssociatedSku' if you want to add some SKUs to an existing product. For example, an existing product (Maxi dress) with 3 SKUs (for size S, M, L). SellerSkus are dress-001, dress-002, dress-003.
You can add SKU via the CreateProduct API by specifying dress-001, dress-002, or dress-003 in the tag.
The content of the 'Attributes" fields are dynamic. To view all available attributes, call the GetCategoryAttributes API. The following table provides some examples.
Name | Type | Description |
---|---|---|
name | string | Name of the product as shown to the customers. Mandatory. Must be between 2 to 255 characters. If this attribute in SPU you used, it becomes not required. |
description | string | Description of the product, as shown to the customers (6 to 25000 characters). Certain HTML tags are supported, but must be escaped as character data (see Guide for Creating Products for the list of supported HTML tags]). Optional |
short_description | string | Highlights of the product. Mandatory. If this attribute in SPU you used, it becomes not required. |
brand | string | Brand name of the product. Mandatory. If this attribute in SPU you used, it becomes not required. |
model | string | The model name of the product. Optional |
warranty | string | The warranty time for the product. Optional |
warranty_type | string | The warranty type of the product. Optional |
color_family | string | Color family. Optional |
... | Other attributes defined in the PrimaryCategory. |
An SKU contains the following tags.
Name | Type | Description |
---|---|---|
SellerSku | string | A unique identifier for the product within the Seller Center instance that is to be added to the system. This identifier is usually freely assigned. Harmonized identifiers, such as UPC or EAN can be set via ProductId. Mandatory |
price | decimal | The product price. Not really a Double, but a Decimal. Mandatory |
quantity | integer | The current level of inventory for this product. Optional |
special_price | decimal | The (hopefully reduced) price for the product while it is on sale. If special_price is specified, either special_from_date or special_to_date must be given; vice versa, if at least one of special_from_date or special_to_date is specified, special_price is mandatory. Not really a Double, but a Decimal. |
special_from_date | datetime | Time and date when the product goes on sale. If passed in, special_price becomes mandatory. The value of 'Time' is accepted in intervals of 15 mins. (For ex: 2017-07-15 18:23 will be converted to 2017-07-15 18:15) |
special_to_date | datetime | Time and date when the sale of the product ends. If passed in, special_price becomes mandatory. The value of 'Time' is accepted in intervals of 15 mins. (For ex: 2017-07-15 18:23 will be converted to 2017-07-15 18:15) |
package_height | string | Package height. Mandatory |
package_length | string | Package length. Mandatory |
package_width | string | Package width. Mandatory |
package_weight | string | Package weight. Mandatory |
package_content | string | Package Content. Optional |
Images | subsection | Contains most 8 images URL. Optional |
Image | string | The image URL. You can set at most 8 images for an SKU. The first image will be used as MainImage. Optional. Use the UploadImages or MigrateImages call to get the image URLs. This will ensure the success rate of creating products. |
Request body
<?xml version="1.0" encoding="UTF-8" ?>
<Request>
<Product>
<PrimaryCategory>6614</PrimaryCategory>
<SPUId></SPUId>
<AssociatedSku></AssociatedSku>
<Attributes>
<name>api create product test sample</name>
<short_description>This is a nice product</short_description>
<brand>Remark</brand>
<model>asdf</model>
<kid_years>Kids (6-10yrs)</kid_years>
</Attributes>
<Skus>
<Sku>
<SellerSku>api-create-test-1</SellerSku>
<color_family>Green</color_family>
<size>40</size>
<quantity>1</quantity>
<price>388.50</price>
<package_length>11</package_length>
<package_height>22</package_height>
<package_weight>33</package_weight>
<package_width>44</package_width>
<package_content>this is what's in the box</package_content>
<Images>
<Image>http://sg.s.alibaba.lzd.co/original/59046bec4d53e74f8ad38d19399205e6.jpg</Image>
<Image>http://sg.s.alibaba.lzd.co/original/179715d3de39a1918b19eec3279dd482.jpg</Image>
</Images>
</Sku>
<Sku>
<SellerSku>api-create-test-2</SellerSku>
<color_family>Green</color_family>
<size>41</size>
<quantity>2</quantity>
<price>520.88</price>
<package_length>11</package_length>
<package_height>22</package_height>
<package_weight>33</package_weight>
<package_width>44</package_width>
<package_content>this is what's in the box</package_content>
<Images>
<Image>http://sg.s.alibaba.lzd.co/original/59046bec4d53e74f8ad38d19399205e6.jpg</Image>
<Image>http://sg.s.alibaba.lzd.co/original/179715d3de39a1918b19eec3279dd482.jpg</Image>
</Images>
</Sku>
</Skus>
</Product>
</Request>
//CreateProduct requires three piece of information
//1.Category Id --- which can be queried by GetCategoryTree
//2.Product attributes --- whose key set and value set can be queried by GetCategoryAttributes
//3.one or more Sku(s) attributes --- the set and value set can be queried by GetCategoryAttributes
//construct attributes of product
Map<String, Object> attributes = new HashMap<String, Object>();
attributes.put("warranty_type", "No Warranty");
attributes.put("package_height", 11.1);
attributes.put("short_description", "yucheng CreateProduct test");
attributes.put("name", "yucheng's first product");
attributes.put("name_ms", "yucheng's first product");
attributes.put("model", "test model");
attributes.put("brand", "Huawei");
attributes.put("display_size_mobile", "4.3");
attributes.put("operating_system_version", "Android 5.1 Lollipop");
attributes.put("operating_system","Android");
//construct SKUs
List<Map<String, Object>> skusList = new ArrayList<>();
Map<String, Object> sku1 = new HashMap<String, Object>();
sku1.put("SellerSku", "yucheng-test-sku-2017020305");
sku1.put("package_height", 11.1);
sku1.put("short_description", "yucheng CreateProduct test");
sku1.put("package_width", 5.9);
sku1.put("tax_class", "default");
sku1.put("package_content", "empty");
sku1.put("package_weight", 11.9);
sku1.put("package_length", 19.3);
sku1.put("color_family", "Blue");
sku1.put("storage_capacity_new", "128G");
skusList.add(sku1);
Map<String, Object> sku2 = new HashMap<String, Object>();
sku2.put("SellerSku", "yucheng-test-sku-2017020306");
sku2.put("package_height", 11.1);
sku2.put("short_description", "yucheng CreateProduct test");
sku2.put("package_width", 5.9);
sku2.put("tax_class", "default");
sku2.put("package_content", "empty");
sku2.put("package_weight", 11.9);
sku2.put("package_length", 19.3);
sku2.put("color_family", "Blue");
sku2.put("storage_capacity_new", "64GB");
skusList.add(sku2);
//construct request by categoryId, attributes of product, attributes of sku(s)
CreateProduct createProduct = new CreateProduct(3L, attributes, skusList);
try {
ModifyProductResponse response = createProduct.execute();
System.out.println(String.format("CreateProduct succeeded?%b",response.getBody()));
} catch (LazadaException e) {
System.out.println(e.getResponseStr());
}
//this is file for put lazada api xml format
$tmpFile = 'test.xml';
///SENDING DATA TO LAZADA API
$curl = curl_init();
//TRUE to HTTP PUT a file. The file to PUT must be set with CURLOPT_INFILE and CURLOPT_INFILESIZE.
curl_setopt( $curl, CURLOPT_PUT, 1 );
//display headers
curl_setopt( $curl, CURLOPT_HEADER, true);
//The name of a file holding one or more certificates to verify the peer with. This only makes sense when used in combination with CURLOPT_SSL_VERIFYPEER.
curl_setopt( $curl, CURLOPT_SSL_VERIFYPEER, false);
// A directory that holds multiple CA certificates. Use this option alongside CURLOPT_SSL_VERIFYPEER.
curl_setopt( $curl, CURLOPT_SSL_VERIFYHOST, false);
// TRUE to HTTP PUT a file. The file to PUT must be set with CURLOPT_INFILE and CURLOPT_INFILESIZE.
curl_setopt( $curl, CURLOPT_INFILESIZE, filesize($tmpFile) );
//The expected size, in bytes, of the file when uploading a file to a remote site.
curl_setopt( $curl, CURLOPT_INFILE, ($in=fopen($tmpFile, 'r')) );
//A custom request method to use instead of "GET" or "HEAD" when doing a HTTP request.
curl_setopt( $curl, CURLOPT_CUSTOMREQUEST, 'POST' );
//An array of HTTP header fields to set,
curl_setopt( $curl, CURLOPT_HTTPHEADER, [ 'Content-Type: application/x-www-form-urlencoded' ] );
//The URL to fetch.
curl_setopt( $curl, CURLOPT_URL, $target );
//TRUE to return the transfer as a string of the return value of curl_exec() instead of outputting it out directly.
curl_setopt( $curl, CURLOPT_RETURNTRANSFER, 1 );
//executing code for connection
$result = curl_exec($curl);
//closing connection
curl_close($curl);
//closing the open file CURLOPT_INFILE
fclose($in);
//printing data for user
print $result;
Result sample
A success example is as follows.
<?xml version="1.0" encoding="UTF-8"?>
<SuccessResponse>
<Head>
<RequestId/>
<RequestAction>CreateProduct</RequestAction>
<ResponseType>Product</ResponseType>
<Timestamp>2016-07-06T20:12:14+0700</Timestamp>
</Head>
<Body/>
</SuccessResponse>
{
"SuccessResponse": {
"Head": {
"RequestId": "",
"RequestAction": "CreateProduct",
"ResponseType": "Product",
"Timestamp": "2016-08-26T06:57:26+0000"
},
"Body": {
"Warnings": []
}
}
}
A failure example is as follows.
<?xml version="1.0" encoding="UTF-8"?>
<ErrorResponse>
<Head>
<RequestAction>CreateProduct</RequestAction>
<ErrorType>Platform</ErrorType>
<ErrorCode>500</ErrorCode>
<ErrorMessage>E500: Create product failed</ErrorMessage>
</Head>
<Body>
<Errors>
<ErrorDetail>
<Field>SellerSku</Field>
<Message>[SELLER_SKU_IS_EXIST]</Message>
</ErrorDetail>
</Errors>
</Body>
</ErrorResponse>
{
"ErrorResponse": {
"Head": {
"RequestAction": "CreateProduct",
"ErrorType": "Platform",
"ErrorCode": 500,
"ErrorMessage": "E500: Create product failed"
},
"Body": {
"Errors": [
{
"Field": "SellerSku",
"Message": "[SELLER_SKU_IS_EXIST]"
}
]
}
}
}
Error messages
Error code | Message |
---|---|
1 | E001: Parameter %s is mandatory |
5 | E005: Invalid Request Format |
6 | E006: Unexpected internal error |
30 | E030: Empty Request |
201 | E201: %s Invalid CategoryId |
202 | E202: %s Invalid SPUId |
205 | E205: SPU not exist |
206 | E206: Different category id in SPU and PrimaryCategory |
500 | E500: Create product failed |
502 | E502: Search SPU failed |
901 | E901: The request is too frequent, or the requested functionality is temporarily disabled. |
1000 | Internal Application Error |
Updated over 6 years ago